Hello,
i'm using frida to test a call to a function located in a class that is not "normaly used" by the game (i think is used by developer for testing purpose).
The class is like so:
i am able to create a pointer to "EnableInvincibility()" and call it successfully and its works, same for "KillMonster()", however, i got an "access violation accessing 0x10" exception when calling Gold(amount).
It happens basically for all function that require a parameter. Basically this error means program try to access a memory address "0x10" which is obviously a wrong address. To try to fix this, i allocated memory, write the (long)amount value inside it, and give that pointer to the function as param, but result is the same.
The 'Gold(long amount) function in IDA looks like so:
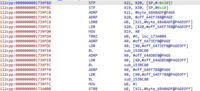
Do you guys have some hints ?
Thanks a lot
i'm using frida to test a call to a function located in a class that is not "normaly used" by the game (i think is used by developer for testing purpose).
The class is like so:
C#:
public class ConsoleCommand // TypeDefIndex: 1447
{
// Fields
private static bool _enableFPS; // 0x0
private static GameObject _fpsObj; // 0x8
// Methods
// RVA: 0x14FDEEE Offset: 0x14FDEEE VA: 0x14FDEEE
public static void RegisterCommandHandlers() { }
[CommandHandler]
// RVA: 0x1500764 Offset: 0x1500764 VA: 0x1500764
public static void EnableInvincibility() { }
[CommandHandler]
// RVA: 0x14FE37F Offset: 0x14FE37F VA: 0x14FE37F
public static void KillMonster() { }
[CommandHandler]
// RVA: 0x14FEDD0 Offset: 0x14FEDD0 VA: 0x14FEDD0
public static void Gold(long amount = 1) { }
}
i am able to create a pointer to "EnableInvincibility()" and call it successfully and its works, same for "KillMonster()", however, i got an "access violation accessing 0x10" exception when calling Gold(amount).
It happens basically for all function that require a parameter. Basically this error means program try to access a memory address "0x10" which is obviously a wrong address. To try to fix this, i allocated memory, write the (long)amount value inside it, and give that pointer to the function as param, but result is the same.
The 'Gold(long amount) function in IDA looks like so:
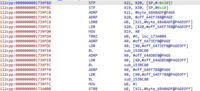
Do you guys have some hints ?
Thanks a lot
Last edited: