Before open this thread I already observe and analyze hooking examples in LGL mod menu. I also read some tutorial here
platinmods.com
platinmods.com
Below is the result of my understanding from those tutorial
Before I ask the real question, I wanna let you know that I'm kinda new to LGL mod menu but I'm not a complete noob. Why? Because I only work with hexpatching before
but some function require me to use hooking instead of patching.
Now come to the question. Where to put my code in main.cpp?
Here I can see there are some hooking example in main.cpp
I can just put my code there right? but I don't think I should put Mshookfunction there too
And how to make the case for my code?
Basic Hooking Tutorial - Platinmods.com - Android & iOS MODs, Mobile Games & Apps
Hey guys, it's SliceCast here. This tutorial is for Advanced Modders who wants to step up their games to get better! Could be for newbies too. I will show you some basics examples on how to hook a function. Now let's say our Type Functions are (int, float, bool, double) and that we're doing a...
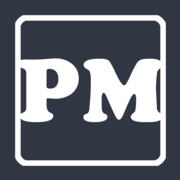
How to unlink functions in IL2CPP and other native games - Platinmods.com - Android & iOS MODs, Mobile Games & Apps
~Read this tutorial first: Basic Hooking Tutorial ~Use this template to do this: LGLTeam/Android-Mod-Menu ~You need some knowledge of C++ to understand this (You can learn C++ on sites such as Geeks For Geeks, TutorialsPoint, Youtube etc. or from apps in the Play Store) Before I start, what do...
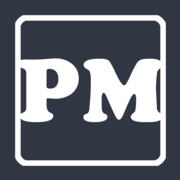
Below is the result of my understanding from those tutorial
C++:
// Token: 0x06000A27 RID: 2599 RVA: 0x00002050 File Offset: 0x00000250
[Token(Token = "0x60007CB")]
[Address(RVA = "0x4D7B38", Offset = "0x4D7B38", VA = "0x4D7B38")]
public void AddCoin(int _amount)
{
}
C++:
int (*old_AddCoin)(void *instance);
int AddCoin(void *instance)
{
if(instance != NULL)
{
return 100100100;
}
return old_AddCoin(instance);
}
MsHookFunction((void*)getAbsoluteAddress(0x4D7B38), (void*)AddCoin, (void**)&old_AddCoin);
but some function require me to use hooking instead of patching.
Now come to the question. Where to put my code in main.cpp?
Here I can see there are some hooking example in main.cpp
C++:
bool feature1, feature2, featureHookToggle, Health;
int sliderValue = 1, level = 0;
void *instanceBtn;
void (*AddMoneyExample)(void *instance, int amount);
bool (*old_get_BoolExample)(void *instance);
bool get_BoolExample(void *instance) {
if (instance != NULL && featureHookToggle) {
return true;
}
return old_get_BoolExample(instance);
}
float (*old_get_FloatExample)(void *instance);
float get_FloatExample(void *instance) {
if (instance != NULL && sliderValue > 1) {
return (float) sliderValue;
}
return old_get_FloatExample(instance);
}
int (*old_Level)(void *instance);
int Level(void *instance) {
if (instance != NULL && level) {
return (int) level;
}
return old_Level(instance);
}
void (*old_Update)(void *instance);
void Update(void *instance) {
instanceBtn = instance;
return old_Update(instance);
}
//Field offset hooking
void (*old_HealthUpdate)(void *instance);
void HealthUpdate(void *instance) {
if (instance != NULL) {
if (Health) {
*(int *) ((uint64_t) instance + 0x48) = 999;
}
}
return old_HealthUpdate(instance);
}
C++:
MsHookFunction((void*)getAbsoluteAddress(0x4D7B38), (void*)AddCoin, (void**)&old_AddCoin);